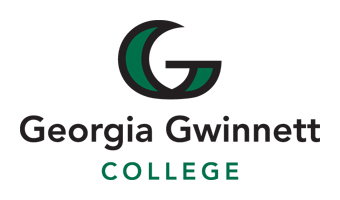
9. Counting
There are three basic counting rules used in this section, one for each of the arithmetic operations of multiplication, addition and subtraction.
9.1. The Product Rule ( and )
To find the total number of outcomes for two or more successive events where both events must occur, multiply the number of outcomes for each event together. For instance, if you want to find the number of outcomes possible when you roll a die and toss a coin, you could use the product rule. It is important to note that the events must be independent, meaning one doesn’t effect the other.
This is called the product rule for counting because it involves multiplying to find a product. The product rule can be extended to more than two choices.
Think of the product rule when you see AND. |
9.2. The Sum Rule ( xor )
Consider finding the total number of ways a particular event occurs from a variety of different options. For instance, if you want to find the number of ways to pick an ITEC major to win a prize knowing there are students from many concentrations, you could use the sum rule. It is important to note the events must be mutually exclusive, meaning they do not have anything in common.
This is called the sum rule for counting because it involves adding to find a total. The sum rule can also be extended to more than two choices.
Think of the sum rule when you see OR. |
Video Example
9.3. The Subtraction Rule ( or )
If your events are not mutually exclusive, to find the total number of possibilities use the subtraction rule.
The subtraction rule is also called the inclusion-exclusion principle.
Video Example
9.4. The Pigeonhole Principle
A suprising number of counting problems can be solved with the so-called pigeonhole principle.
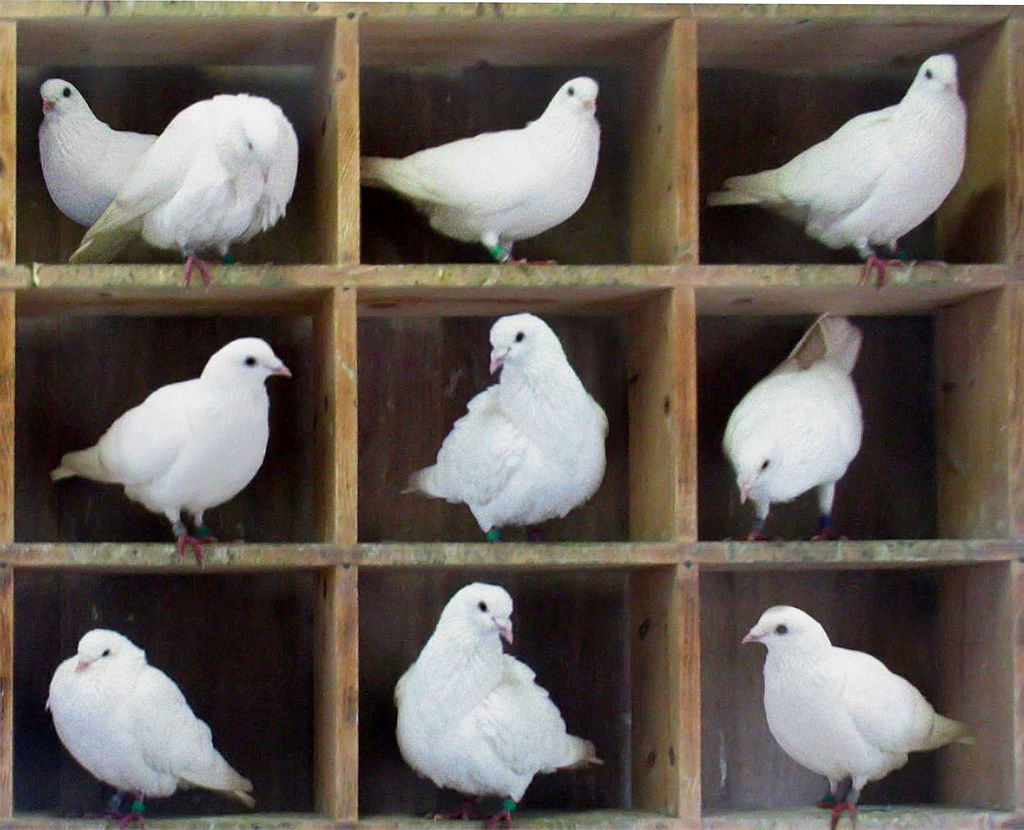
Ten pigeons in nine pigeonholes
9.5. Permutations and Combinations
Consider the first four letters of the alphabet, \(A, B, C, D\). A permutation of these letters would be an arrangement of them in different orders.
-
\(ABCD, BACD, ABDC\) are permutations of the letters taken four at a time
-
\(BDA, ABC, ABD\) are permuations of the letters taken three at a time
-
\(AB, AC, DB\) are permutations of the letters taken two at a time
In general, given a set of \(n\) objects, an ordered arrangment of \(r \le n\) of them is called an \(r\)-permutation or a permutation of \(n\) objects taken \(r\) at a time . We will denote this by \(P(n,r)\). (Note that \(_nP_r\) is a commonly used notation, especially on calculators.)
Video Example
Now consider the letters \(A, B, C, D\) and choose three at a time where the order does not matter. That is, the choice \(ABC\) is the same choice as \(BCA\). This selection where order does not matter is called a combination . There are only four possible ways to choose three letters without regard to order:
*\(ABC, ABD, ACD,\) and \(BCD\).
In general, given a set of \(n\) objects, and unordered selection of \(r\le n\) of them is an \(r\)-combination or a combination of \(n\) objects taken \(r\) at a time . We will denote this by \(C(n,r)\), read as '\(n\) choose \(r\)'. (Note that \(_nC_r\) is commonly used by calculators.)
Video Example
Video Example
9.6. Exercises
-
There are 67 math majors and 124 ITEC majors at a college.
-
In how many ways can two representatives be picked so that one is a math major and one is an ITEC major?
-
In how many ways can one representative be picked who is either a math major or an ITEC major?
-
-
A multiple-choice test contains 20 questions, and each question has four choices.
-
In how many ways can a student answer all of the questions on the test?
-
In how many ways can a student answer all of the questions if she is allowed to leave some blank?
-
-
How many different three-letter initials can a person have?
-
How many different three-letter initials end with "R"?
-
How many bit strings are there of length five?
-
How many bit strings are there of length five that begin and end with 1?
-
How many bit strings are there of length less than \(n\), where \(n\) is a positive integer, that start and end with 1?
-
How many license plates can be made using three digits followed by four uppercase English letters if:
-
Digits and letters can be repeated?
-
Digits and letters cannot be repeated?
-
-
If each student in Discrete Mathematics is a mathematics major, an ITEC major, or a double major, and a class has 5 math majors (including double majors), 23 ITEC majors (including double majors), and 7 double majors, how many students are in the class?
-
Suppose a computer system requires a password of length no less than 7 and no more than 10 characters, and each character must be an English lowercase letter, an English uppercase letter, a digit, or one of six special characters (*, >, <, !, +, =).
-
How many different passwords are available?
-
Suppose a hacker can check a potential password once every nanosecond (1 nanosecond is \(1 \times 10^{-9}\) seconds). How long will it take the hacker to check every potential password?
-
-
Show that if there are 29 students in a class at least two have last names that begin with the same letter.
-
How many students need to be in a class in order for at least two students to receive the same grade on an exam that is graded on a scale 0 to 100 points?
-
Show that in any set of 5 integers, there are at least two of them that have the same remainder when divided by 4.
-
A bag contains 8 red balls and 7 blue balls.
-
How many balls must be chosen to be sure of choosing 3 of the same color?
-
How many must be chosen to be sure of choosing 3 red balls?
-
-
Someone cleaning out their attic finds a box containing 12 rock CDs and 12 country CDs. What is the minimum number of CDs they can take out to guarantee at least one of each type?
-
Give an argument that there are at least two people in Atlanta with the same number of hairs on their head.
-
There are 67 math majors and 124 ITEC majors at a college.
-
In how many ways can two representatives be picked so that one is a math major and one is an ITEC major?
-
In how many ways can one representative be picked who is either a math major or an ITEC major?
-
-
A multiple-choice test contains 20 questions, and each question has four choices.
-
In how many ways can a student answer all of the questions on the test?
-
In how many ways can a student answer all of the questions if she is allowed to leave some blank?
-
-
How many different three-letter initials can a person have?
-
How many different three-letter initials end with "R"?
-
How many bit strings are there of length five?
-
How many bit strings are there of length seven that begin and end with 0?
-
How many bit strings are there of length less than \(n\), where \(n\) is a positive integer, that start and end with 1?
-
How many license plates can be made using four digits followed by three uppercase English letters if:
-
Digits and letters can be repeated?
-
Digits and letters cannot be repeated?
-
-
If each student in Discrete Mathematics is a mathematics major, an ITEC major, or a double major, and a class has 18 math majors (including double majors), 25 ITEC majors (including double majors), and 8 double majors, how many students are in the class?
-
Suppose a computer system requires a password of length no less than 8 and no more than 11 characters, and each character must be an English lowercase letter, an English uppercase letter, a digit, or one of six special characters (*, >, <, !, +, =).
-
How many different passwords are available?
-
Suppose a hacker can check a potential password once every nanosecond (1 nanosecond is \(1 \times 10^{-9}\) seconds). How long will it take the hacker to check every potential password?
-
-
Show that if there are 29 students in a class at least two have last names that begin with the same letter.
-
How many students need to be in a class in order for at least two students to receive the same grade on an exam that is graded on a scale 0 to 50 points?
-
Show that in any set of 8 integers, there are at least two of them that have the same remainder when divided by 7.
-
A bag contains 12 red balls and 7 blue balls.
-
How many balls must be chosen to be sure of choosing 4 of the same color?
-
How many must be chosen to be sure of choosing 4 red balls?
-
-
Someone cleaning out their attic finds a box containing 12 rock CDs, 12 hip hop CDs, and 12 country CDs. What is the minimum number of CDs they can take out to guarantee at least one of each type?
-
Give an argument that there are at least two people in Atlanta with the same number of hairs on their head.
-
List all the permutations of \(\{1, 2, 3\}\).
-
How many permutations are there of the set \(\{1, a, 2, b, 3, c, 5\}\)?
-
Let \(A=\{a, b, c, d\}\)
-
List all the 3-permutations of \(A\).
-
List all the 3-combinations of \(A\).
-
-
Let \(A=\{a, b, c, d, e\}\)
-
List all the 2-permutations of \(A\).
-
List all the 2-combinations of \(A\).
-
-
Find the value of the following
-
\(P(5,2)\)
-
\(P(10,8)\)
-
\(P(14,10)\)
-
\(P(12,8)\)
-
\(C(5,2)\)
-
\(C(10,8)\)
-
\(C(14,10)\)
-
\(C(12,8)\)
-
-
How many bit strings of length 10 contain:
-
Exactly five 1s?
-
At most five 1s?
-
At least four 1s?
-
The same number of 0s and 1s?
-
-
How many permutations of the digits \(12345678\) contain:
-
The string 284?
-
The string 3581?
-
The string 21 and 57?
-
-
How many ways are there to choose 9 cards from a standard 52 card deck?
-
How many ways can you be dealt a pair in a 5 card hand (2 cards of the same rank and 3 cards of a different rank)?
-
How many ways can you be dealt a full house in a 5 card hand (2 cards of the same rank and 3 cards of the same rank)?
-
How many license plates consist of 4 letters followed by 3 digits if:
-
Repetition is allowed?
-
Repetition is not allowed?
-
-
Using \(C(n,r) = \displaystyle \frac{n!}{r!(n-r)!}\), evaluate the terms of this triangular table. Will you need the formula to extend the table to more rows?
\begin{array}{ccccccccccccc} &&&&&&&C(0,0)&&&&&&\\ &&&&&& C(1,0) && C(1,1) &&&&&\\ &&&&& C(2,0) && C(2,1) && C(2,2) &&&&\\ &&&& C(3,0) && C(3,1) && C(3,2) && C(3,3) &&&\\ &&& C(4,0) && C(4,1) && C(4,2) && C(4,3) && C(4,4) &&\\ \end{array}